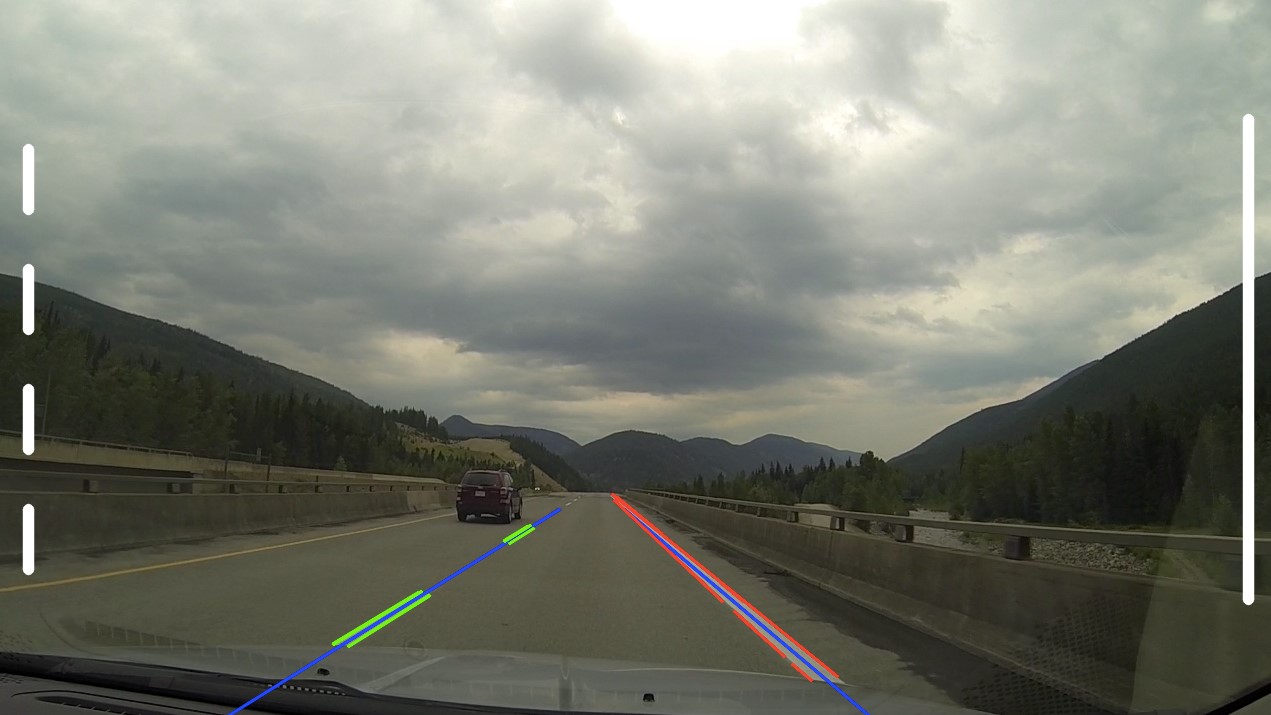
Michal Polko
In this project, we detect lane markers in videos taken with dashboard camera.
Process
- Convert a video frame to grayscale, boost contrast and apply dilation operator to highlight lane markers in the frame.
Highlighted lane markers. cvtColor(frame, frame_bw, CV_RGB2GRAY); frame_bw.convertTo(frame_bw, CV_32F, 1.0 / 255.0); pow(frame_bw, 3.0, frame_bw); frame_bw *= 3.0; frame_bw.convertTo(frame_bw, CV_8U, 255.0); dilate(frame_bw, frame_bw, getStructuringElement(CV_SHAPE_RECT, Size(3, 3)));
- Apply the Canny edge detection to find edges.
Application of the Canny edge detection. int cny_threshold = 100; Canny(frame_bw, frame_edges, cny_threshold, cny_threshold * 3, 3);
- Apply the Hough transform to find line segments.
vector<Vec4i> hg_lines; HoughLinesP(frame_edges, hg_lines, 1, CV_PI / 180, 15, 15, 2);
- Since the Hough transform returns all line segments, not only those around lane markers, it is necessary to filter the results.
- We create two lines that describe boundaries of the current lane (hypothesis).
- We place two converging lines in the frame.
- Using brute-force search, we try to find position where they capture as many line segments as possible.
- Since road in the frame can have more than one lane, we try to find result as narrow as possible.
- We select line segments that are captured by the created hypothesis, mark them as lane markers and draw them.
- Each frame, we take the detected lane markers from the previous frame and perform linear regression to adjust the hypothesis (continuous adjustment).
- If we cannot find lane markers in more than 5 successive frames (due to failure of continuous adjustment, lane change, intersection, …), we create a new hypothesis.
- If the hypothesis is too wide (almost full width of the frame), we create a new one, because arrangement of road lanes might have changed (e.g. additional lane on freeway).
- We create two lines that describe boundaries of the current lane (hypothesis).
- To distinguish between solid and dashed lane markers, we calculate coverage of the hypothesis by line segments. If the coverage is less than 60%, it is a dashed line; if more, it is a solid line.
Filtered result of the Hough transform + detection of solid/dashed lines.