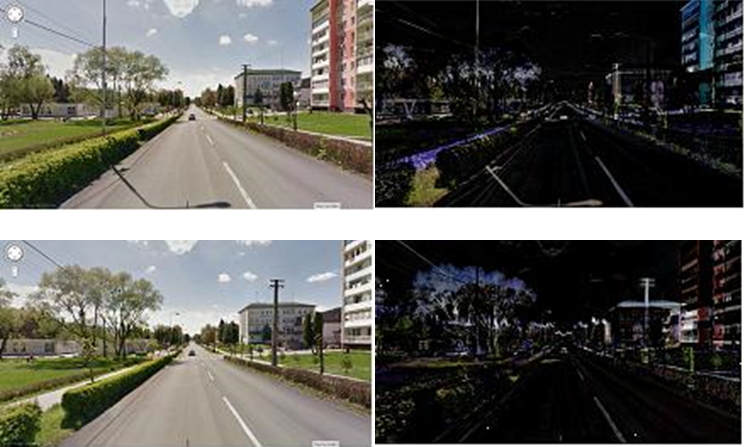
Description
The goal of this project is to create a program that will be able to stitch a sequence of images fromgoogle street-view and make movie from it. The idea came to my mind, when I needed to check thecrossroads and traffic signals along the route I’ve never driven before. The method was tointerpolatefew more images between two consecutive views to simulate moving car. To do that Ihad to do following steps:
Process
- Remove UI elements from images
- Find homography between following images
- Interpolate homography between them
- Put images into movie
Removing UI elements from images
Removing UI elements is important because in later steps I will need to find similar areas and those elements can spoil the match-up. First I cycled through all images and gained areas with same color in black. The resulting image was accumulated from all the differences. Black areas represent pixels that were same in all images. To improve the mask I inverted the image did some thresholding, Gaussian blur and again thresholding. Result was mask used for inpaint method to fill in those regions without UI elements with color.
Example of process
Finding homography
Homography found between two images was found using SURF detector. I improved the detection by using mask of similar areas as in previous step. I did it because many key-points were detected on sky or far objects and results were generally worse. Last step was to interpolate from one image to another using homography. In my program I used 25 steps between two pictures. Those pictures were stacked into movie and saved.
Mat homo = findMatch(pic1, pic2); bj_corners[0] = cvPoint(0,0) ////-- Get the corners from the image_1 ( the object to be "detected" ) vector<Point2f> obj_corners(4); obj_corners[0] = cvPoint(0,0); obj_corners[1] = cvPoint( pic2.cols, 0 ); obj_corners[2] = cvPoint( pic2.cols, pic2.rows ); obj_corners[3] = cvPoint( 0, pic2.rows ); vector<Point2f> corners(4); vector<Point2f> inter_corners(4); perspectiveTransform( obj_corners, corners, homo); ... ... for(int i=0; i<4; i++) { inter_corners[i].x = corners[i].x - distance[i].x*j; inter_corners[i].y = corners[i].y - distance[i].y*j; } Mat interHomo = findHomography( corners, inter_corners, 0 ); Mat transformed; warpPerspective(pic1, transformed, interHomo, Size(600,350)); result[j] = transformed;